Orders of Growth
Order |
Linear |
Polynomial |
Exponential |
Factorial |
Example |
n |
n2, n3 |
2n, en, 10n |
n!, nn |
n=1000 |
103 |
106, 109 |
|
|
Exercise:
For each of the following pairs of functions, indicate which one has a higher order of growth.
- n(n+1) and 2000n2
- ≈ same -- within constant multiple
- 100n2 and 0.01n3
- cubic has higher order of growth than quadratic
- log2n and lnn
- All logarithmic functions have ≈ same growth within const multiple (can change log's base by the formula logan=logablogbn)
- log22n and log2n2
- log22n=log2nlog2n and log2n2=2log2n
- 2n−1 and 2n
- 2n−1=212n so ≈ same order of growth as 2n
- (n−1)! and n!
- (n−1)! has lower order of growth since n!=n(n−1)!
Analysis of Non-Recursive Algorithms
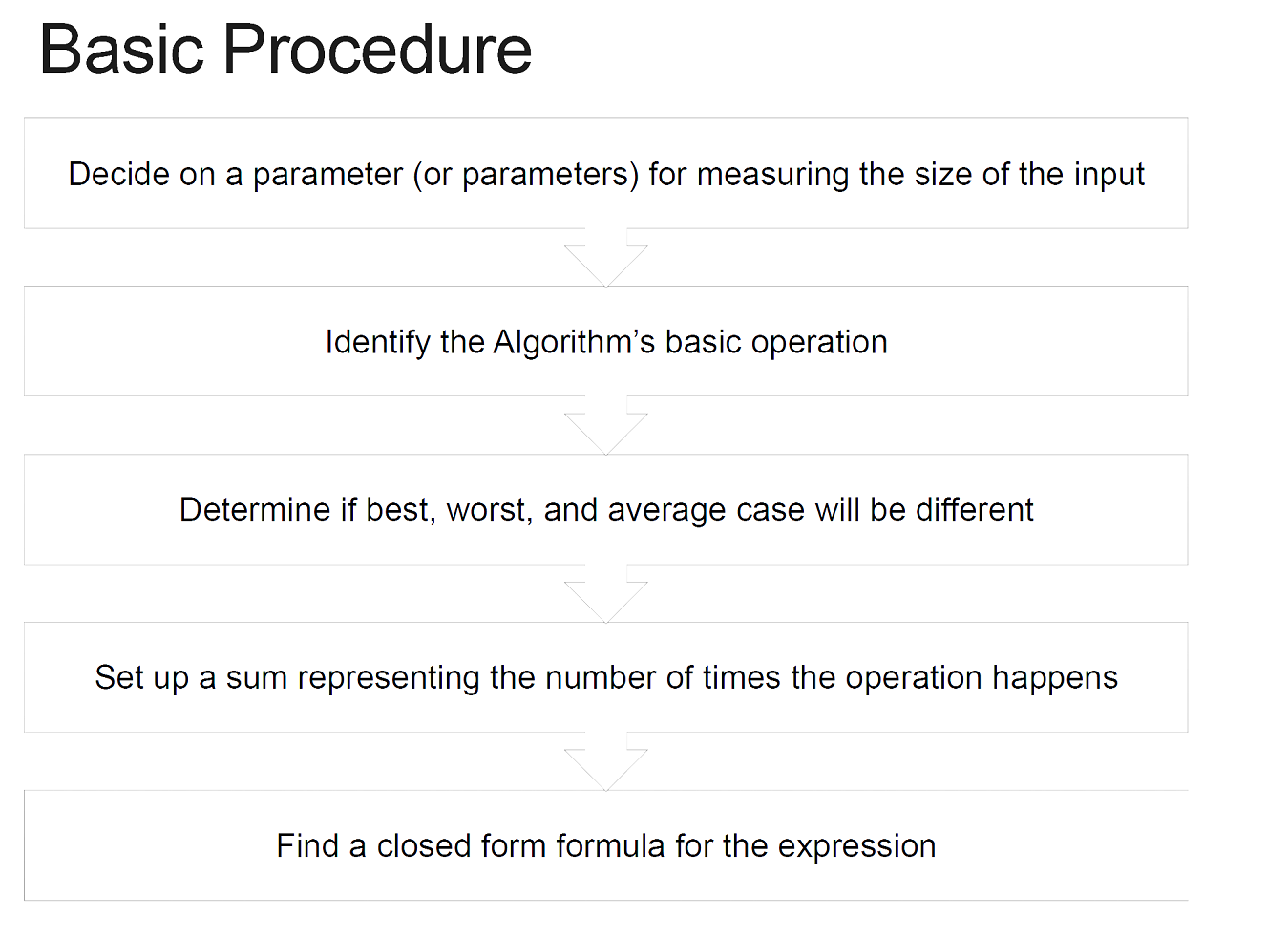
Example 1:
Max Element
Input: an array, A
Output: the largest element in A
maxVal <- A[0]
for i <- 1 ... A.length-1
if A[i] > maxVal
maxVal <- A[i]
return maxVal
What is the input size? (A.length)
What is the basic operation? (Comparison)
What do we expect its complexity class to be? (Linear)
C(n)=∑i=1n−11
=(n−1)−1+1 (from previous formula)
=(n−1)∈Θ(n)
Example 2:
Bubble Sort
Input: an array, A of sortable elements
Output: a permutation of A in non-decreasing order
for i <- 0 ... A.length - 2
for j <- 0 ... A.length - 2 - i
if A[j+1] < A[j]
swap A[j] and A[j+1]
return A
What's the input size? n = A.length
Basic operation? Swap
What do we expect its complexity class to be? Polynomial
C(n)=∑i=0n−2∑j=0n−2−i1
=∑i=0n−2n−1−i (the inner cycles happen n - i - 1 times)
=∑i=1n−1i
=2n(n−1)∈Θ(n2)
Example 3:
Matrix Summation
Input: a w×h matrix, M
Output: The sum of all elements in M
total <- 0
for i <- 0 ... w - 1
for j <- - ... h - 1
total <- total + M[i][j]
return total
Input size? w⋅h
Basic operation? Addition
Expected complexity class? Linear
C(n)=∑i=0w−1∑j=0h−11
=∑i=0w−1h
=h∑i=0w−11
=h⋅w =n∈Θ(n)
Example 4:
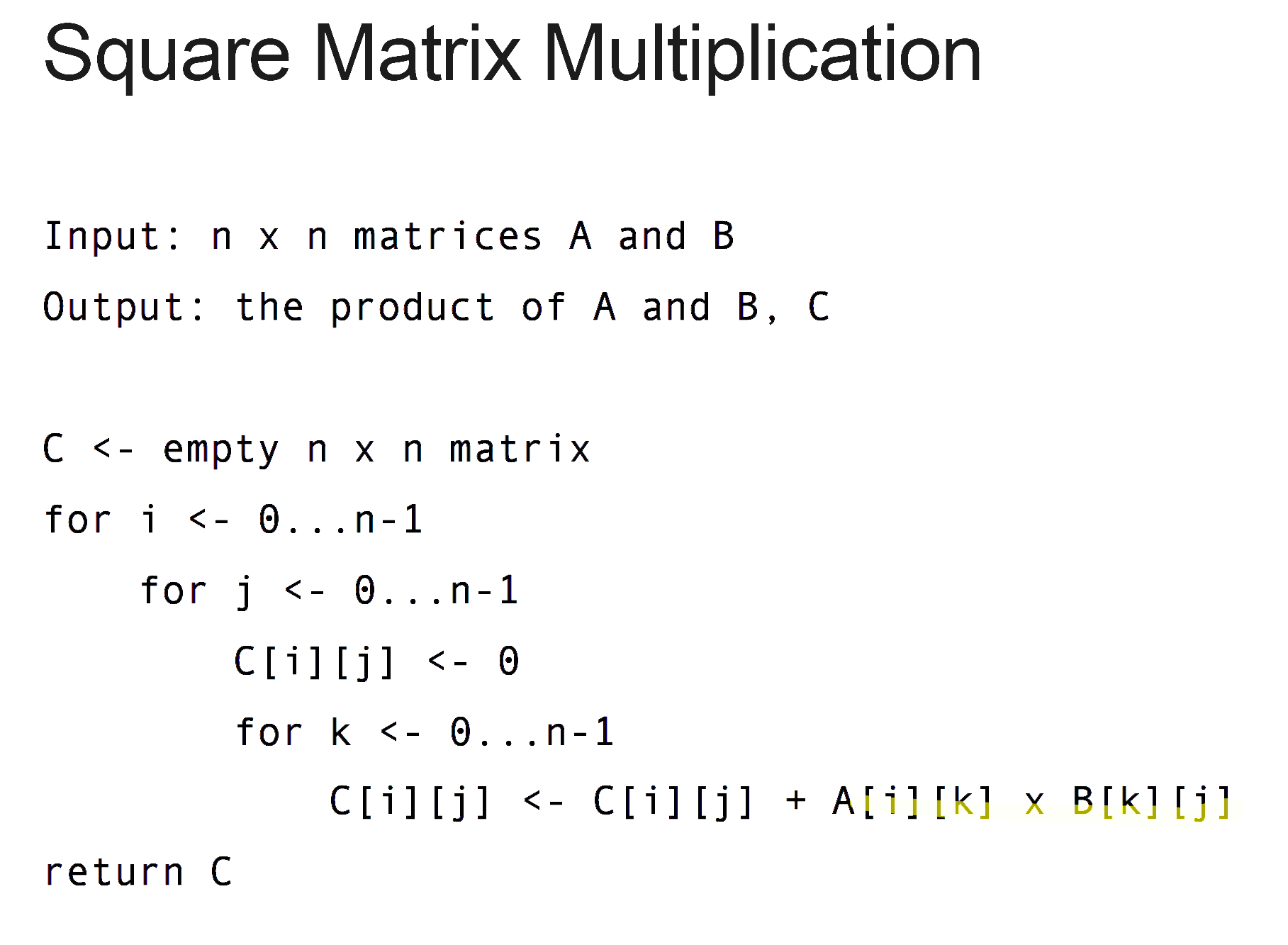
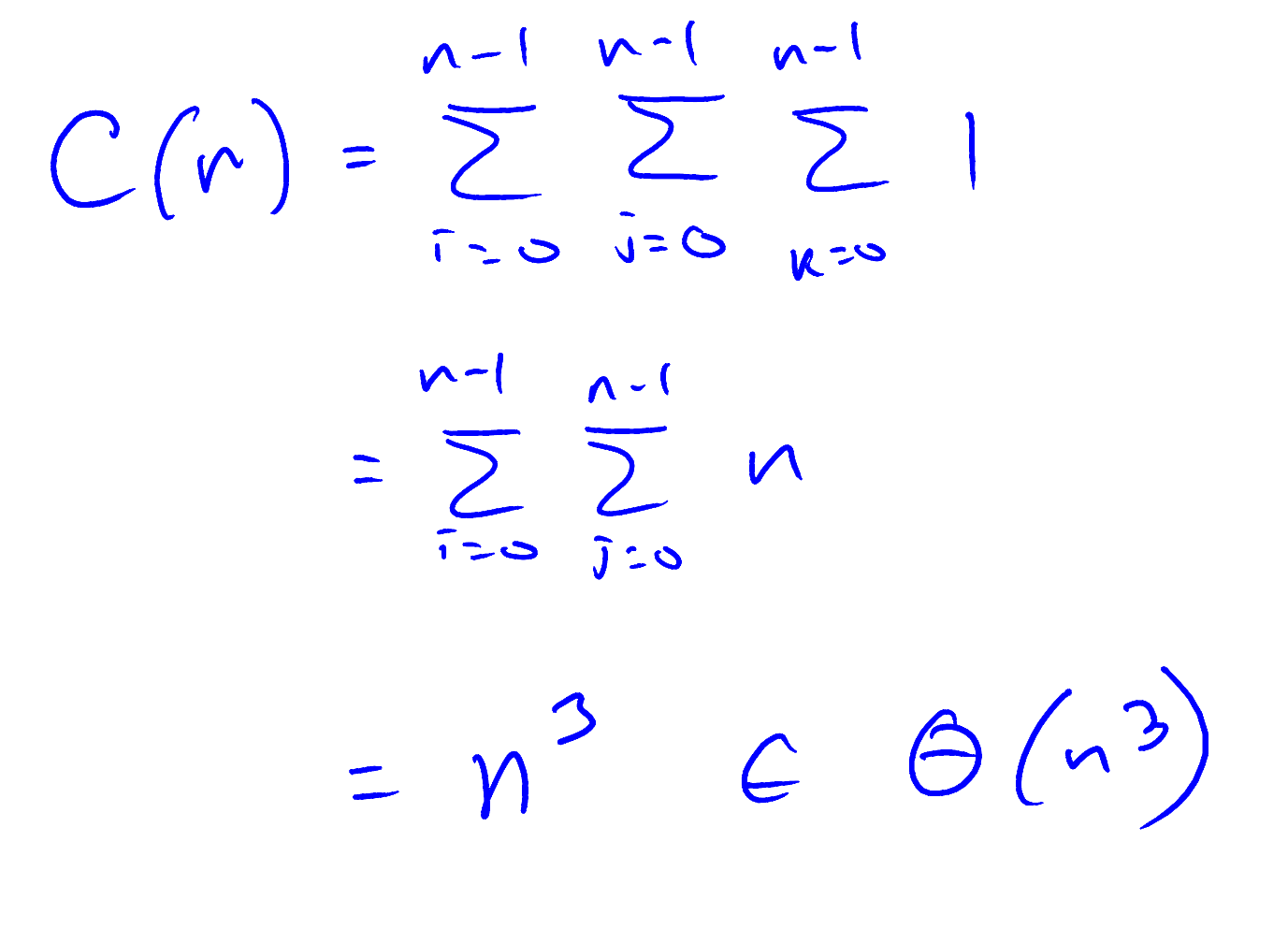
Example 5:
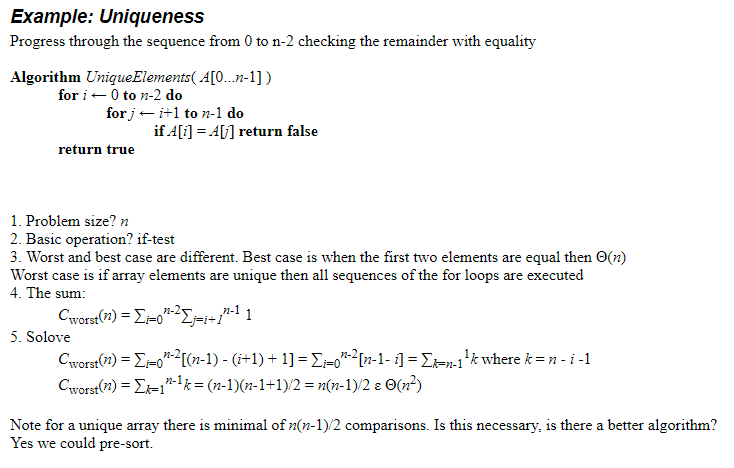